Utility Console Methods For Debugging Your Javascript Workflow
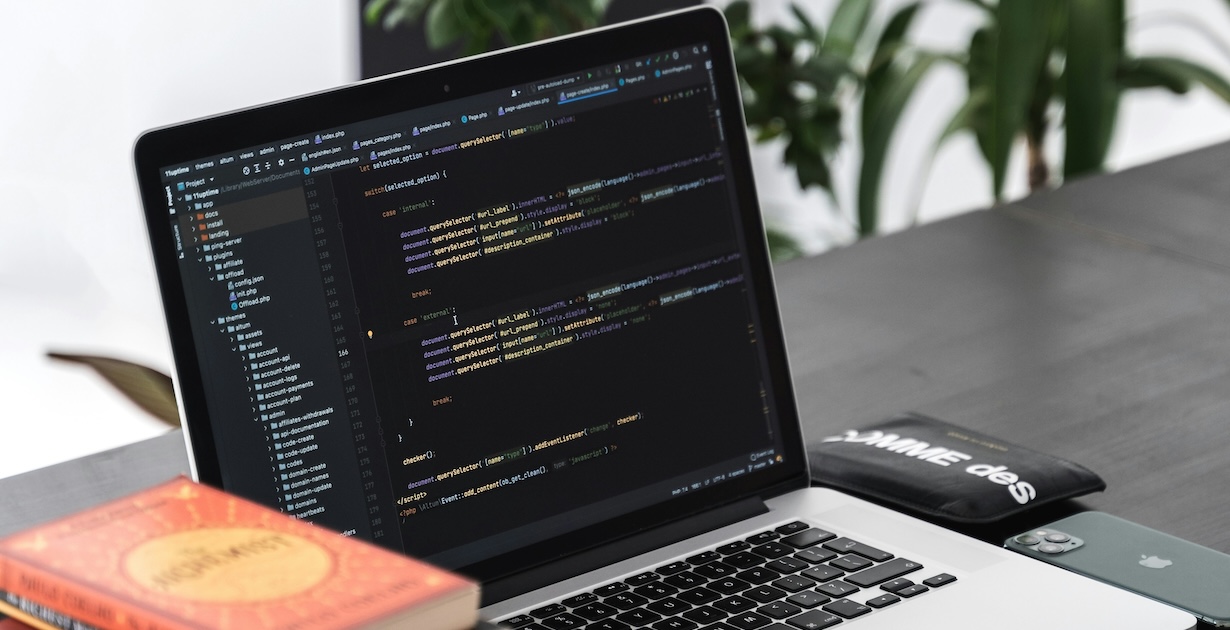
If you've missed the previous parts of this series, you can find Part 1 here and Part 2 here.
This final part of the series which all started with console.assert()
. This one covers utility/debugging methods that go beyond simple logging and help in managing and measuring console outputs when you're debugging your code:
- console.clear() - Clearing the console
- console.time() and console.timeEnd() - Measuring execution time
- console.count() and console.countReset() - Counting occurrences
console.clear();
// Clearing the console
console.log("This will be cleared");
console.clear();
// Console gets cleared, no output visible
console.time();
and console.timeEnd();
// Measuring execution time
console.time("Timer");
for(let i = 0; i < 1000000; i++) {
// Simulate a process
}
console.timeEnd("Timer");
Timer: 2.345ms
console.count();
and console.countReset();
// Counting log occurrences
console.count("Process Count");
console.count("Process Count");
console.countReset("Process Count");
console.count("Process Count");
Process Count: 1
Process Count: 2
Process Count: 1
This is NOT everything. Check out Chromes Console API reference and to delve even deeper, the Chrome DevTools Console Utilities API.