Outputting Git Branches to a CSV
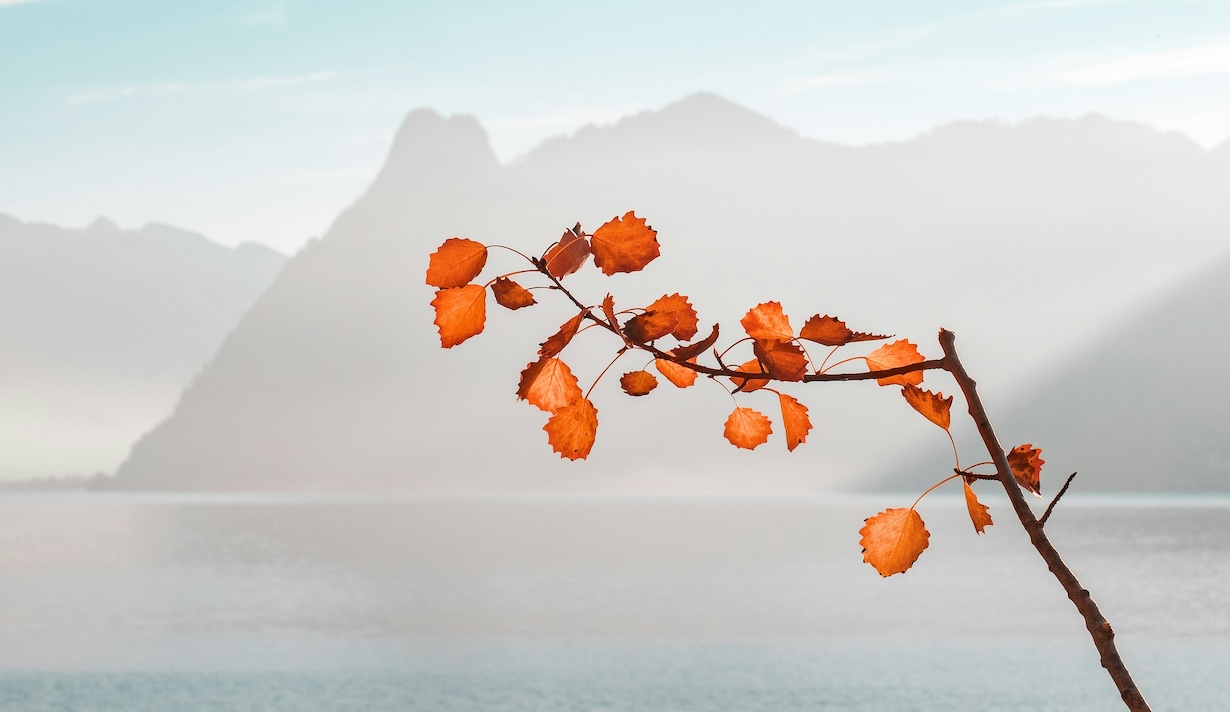
Photo by Simon Berger on Unsplash
This solution isn't perfect, especially if you have commas in your commit messages, but it gets the job done. Initially, I planned to write a quick bash script, but in true developer style, it turned into a 50-line beast. The concept seemed straightforward, like git branch -av >> ~/Downloads/branches.txt
, but I wanted something I could easily import into Excel.
That approach didn't work as well as I hoped, so I thought I'd tweak that one-liner... it's now a lot bigger.
- Run
$ touch export_branches.sh
- Run
$ chmod +x export_branches.sh
$ nano export_branches.sh
and add the following:
#!/bin/bash
# Initialise repo_path and output_csv as empty strings.
repo_path=""
output_csv=""
# Use the getopts command to parse command-line arguments:
# -p for the Git repository path.
# -o for the output CSV file path.
while getopts "p:o:" opt; do
case ${opt} in
p )
repo_path=$OPTARG
;;
o )
output_csv=$OPTARG
;;
\? )
echo "Usage: cmd [-p] [-o] "
exit 1
;;
esac
done
# The script checks if both repo_path and output_csv are provided. If not, it displays an error message and usage information, then exits.
if [[ -z "$repo_path" ]] || [[ -z "$output_csv" ]]; then
echo "Error: Both repository path and output CSV file must be specified."
echo "Usage: $0 -p -o "
exit 1
fi
# The script checks if the specified path is a valid directory and contains a .git folder to confirm it's a Git repository.
if [ -d "$repo_path" ] && [ -d "$repo_path/.git" ]; then
# Navigate to the directory
cd "$repo_path" || exit
# Captures the output of git branch -av, processes it, and writes the results to the specified CSV file.
git branch -av > branches.txt
# Process the output and write to the specified CSV file.
echo "Branch,Commit,Message" > "$output_csv"
while IFS= read -r line; do
branch=$(echo "$line" | awk '{print $1}')
commit=$(echo "$line" | awk '{print $2}')
message=$(echo "$line" | cut -d' ' -f 3-)
echo "$branch,$commit,$message" >> "$output_csv"
done < branches.txt
# The script removes the temporary branches.txt file.
rm branches.txt
# Success output
echo "Branches have been exported to $output_csv"
else
# Something went wrong output
echo "The specified directory does not exist or is not a Git repository."
exit 1
fi
To run, simply call:
./export_branches.sh -p /path/to/git/repository -o /path/to/output/branches.csv