Basic Javascript Console Logging Techniques Every Developer Should Know
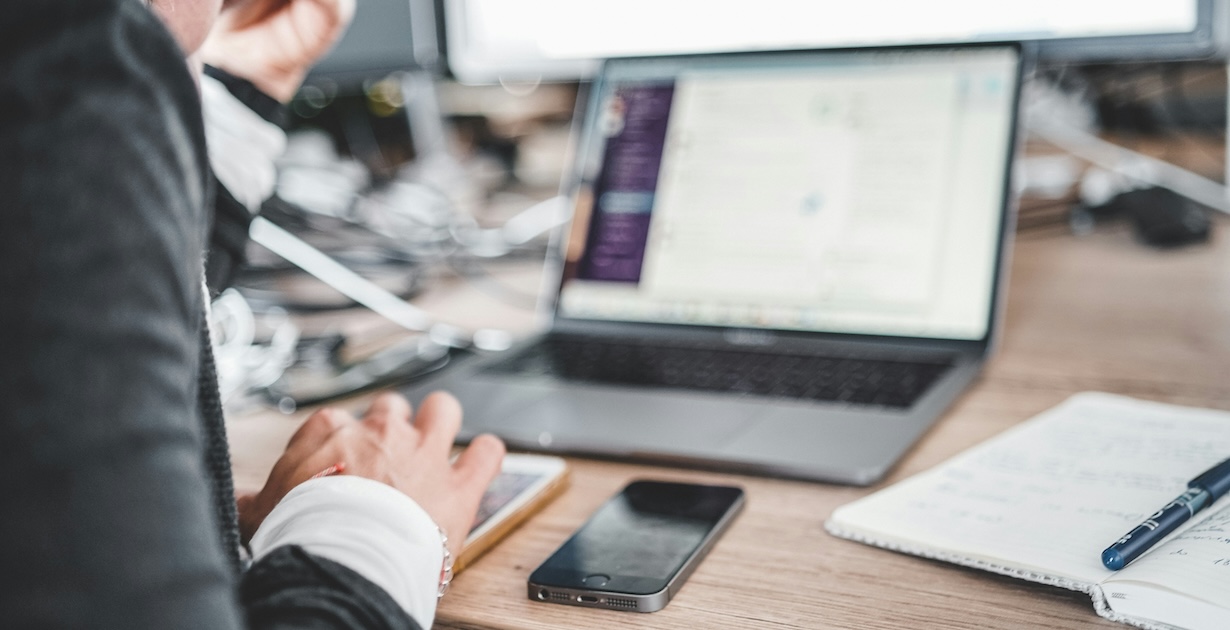
Photo by Zan Lazarevic on Unsplash
In JavaScript, the console object provides access to the browser's debugging console. There are more methods available on the console object than I ever realised or looked into. Over the next few posts, I hope to list them all out along with some examples.
FYI: Part 2 is now out and Part 3.
These are the ones that you should all know
console.log();
// General logging and the basics
let someVar = 42;
console.log(someVar); // Direct output of variables
console.log("This is a log message"); // Strings
console.log(`The answer is ${someVar}`); //Embedded Expression allowed too
console.log("Variable value:", someVar);
Output:
42
This is a log message
The answer is 42
Variable value: 42
console.error();
// Logging errors
console.error("This is an error message");
console.error("Error details:", new Error("Something went wrong"));
Output:
This is an error message
Error details: Error: Something went wrong
at <anonymous>:1:42
console.warn();
// Logging warnings
console.warn("This is a warning message");
console.warn("Warning details:", "Low disk space");
Output:
This is a warning message
Warning details: Low disk space
console.info();
// Logging informational messages
console.info("This is an informational message");
console.info("Info details:", { status: "OK" });
Output:
This is an informational message
Info details: { status: "OK" }
console.debug();
// Logging debug messages
console.debug("This is a debug message");
console.debug("Debug details:", { debugMode: true });
Output:
This is a debug message
Debug details: { debugMode: true }
Why not try it out now in the developer console now? If you're on Chrone/Edge check out this guide.